How Developers Can Enhance Their Debugging Competencies By Gustavo Woltmann
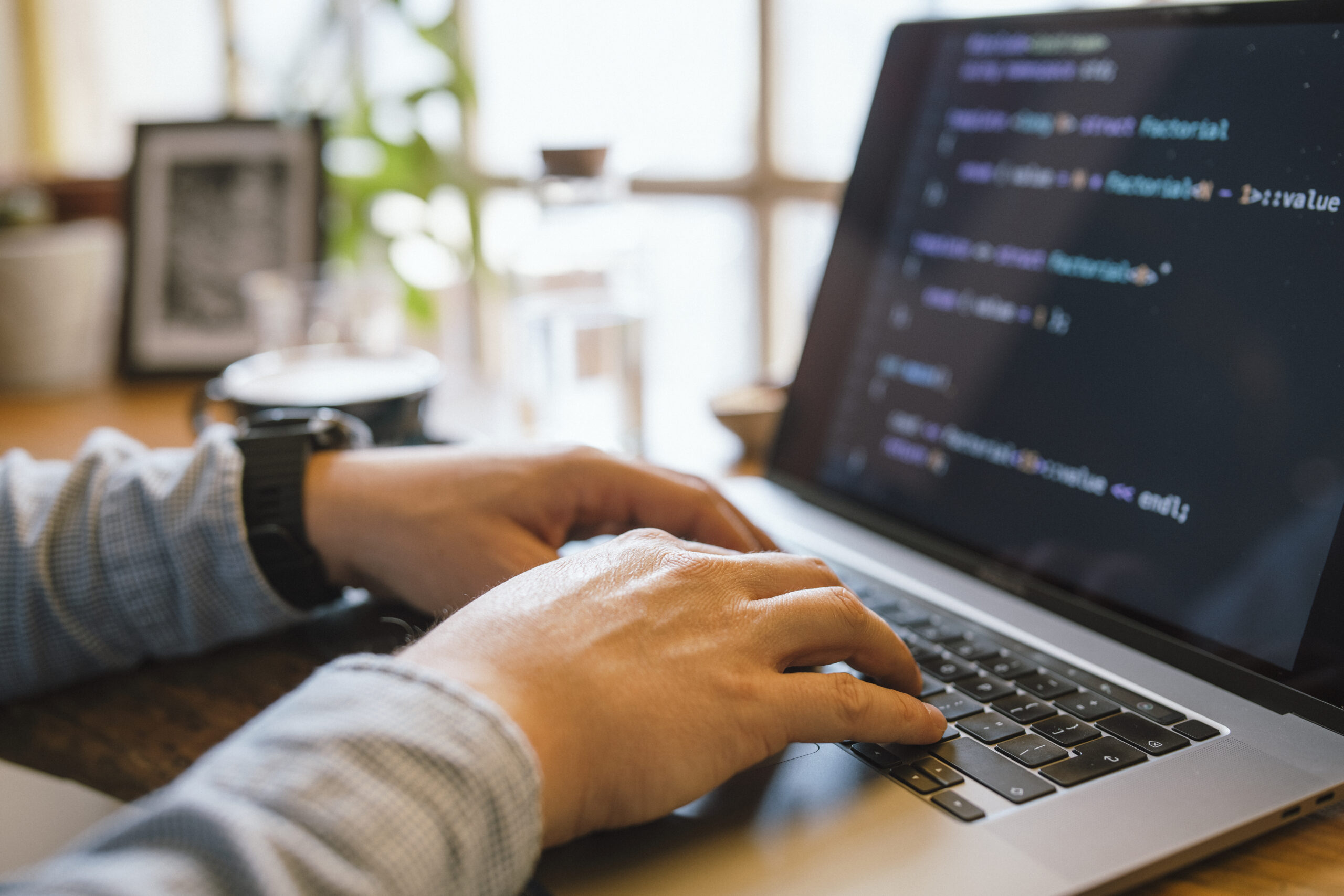
Debugging is Just about the most necessary — yet usually neglected — competencies inside of a developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about comprehending how and why items go Mistaken, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save several hours of annoyance and considerably improve your efficiency. Here i will discuss quite a few procedures that can help builders degree up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of several quickest ways builders can elevate their debugging abilities is by mastering the tools they use every day. Though producing code is one particular Portion of growth, realizing how to connect with it properly in the course of execution is equally important. Fashionable growth environments arrive Geared up with strong debugging capabilities — but lots of builders only scratch the surface area of what these tools can perform.
Get, for example, an Built-in Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, step by code line by line, and also modify code over the fly. When utilised correctly, they Enable you to observe exactly how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for entrance-end developers. They allow you to inspect the DOM, keep an eye on community requests, see authentic-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can transform aggravating UI difficulties into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management more than managing procedures and memory administration. Studying these equipment can have a steeper learning curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with version Handle systems like Git to know code historical past, come across the precise instant bugs were being introduced, and isolate problematic alterations.
In the long run, mastering your applications usually means going beyond default settings and shortcuts — it’s about creating an personal familiarity with your enhancement ecosystem so that when problems arise, you’re not lost at nighttime. The higher you already know your instruments, the greater time it is possible to commit resolving the particular challenge in lieu of fumbling by the method.
Reproduce the Problem
Probably the most essential — and sometimes ignored — actions in effective debugging is reproducing the problem. Right before leaping into your code or building guesses, developers require to create a dependable natural environment or circumstance wherever the bug reliably appears. Without having reproducibility, fixing a bug becomes a activity of possibility, usually resulting in wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you can. Request concerns like: What steps resulted in The difficulty? Which setting was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact disorders beneath which the bug happens.
Once you’ve collected enough data, attempt to recreate the situation in your local atmosphere. This might mean inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The problem seems intermittently, think about producing automatic exams that replicate the sting cases or point out transitions involved. These exams don't just assist expose the challenge but also avoid regressions Down the road.
At times, The difficulty could be natural environment-specific — it might transpire only on certain running systems, browsers, or below unique configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It calls for endurance, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. With a reproducible situation, You should utilize your debugging applications more successfully, check prospective fixes securely, and connect extra Evidently with all your workforce or buyers. It turns an summary grievance right into a concrete obstacle — Which’s wherever developers thrive.
Study and Comprehend the Error Messages
Mistake messages tend to be the most respected clues a developer has when some thing goes wrong. Rather than looking at them as discouraging interruptions, builders must study to deal with error messages as direct communications within the process. They typically let you know precisely what occurred, where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking through the message very carefully and in comprehensive. A lot of developers, specially when beneath time pressure, look at the main line and quickly begin earning assumptions. But further within the mistake stack or logs could lie the true root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them to start with.
Break the mistake down into sections. Is it a syntax mistake, a runtime exception, or a logic mistake? Will it level to a selected file and line amount? What module or perform brought on it? These concerns can guideline your investigation and level you towards the responsible code.
It’s also valuable to know the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can drastically accelerate your debugging procedure.
Some glitches are vague or generic, and in All those cases, it’s vital to look at the context wherein the mistake happened. Check connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These typically precede larger sized issues and provide hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Understanding to interpret them the right way turns chaos into clarity, helping you pinpoint concerns more rapidly, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is Among the most impressive applications in a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an application behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach begins with recognizing what to log and at what amount. Prevalent logging degrees incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic info throughout improvement, INFO for typical situations (like prosperous start off-ups), WARN for potential concerns that don’t break the application, Mistake for true difficulties, and FATAL in the event the technique can’t proceed.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your system. Center on essential occasions, state variations, input/output values, and critical final decision factors in your code.
Structure your log messages clearly and continuously. Incorporate context, including timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs let you keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in manufacturing environments where by stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-assumed-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the In general maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To proficiently identify and repair bugs, developers ought to solution the process like a detective solving a mystery. This frame of mind helps break down complicated concerns into workable sections and abide by clues logically to uncover the foundation cause.
Begin by gathering proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect as much relevant data as it is possible to devoid of leaping to conclusions. Use logs, examination situations, and consumer studies to piece collectively a clear image of what’s occurring.
Next, type hypotheses. Request oneself: What could possibly be leading to this conduct? Have any adjustments not too long ago been produced on the codebase? Has this situation transpired prior to under identical instances? The target is usually to slim down prospects and discover prospective culprits.
Then, check your theories systematically. Try to recreate the condition in a very managed atmosphere. If you suspect a selected operate or component, isolate it and verify if The problem persists. Like a detective conducting interviews, ask your code issues and Allow the effects direct you closer to the reality.
Shell out near attention to smaller information. Bugs frequently disguise inside the least predicted locations—similar to a missing semicolon, an off-by-a single mistake, or even a race condition. Be extensive and patient, resisting the urge to patch The problem without thoroughly knowing it. Non permanent fixes could conceal the actual issue, just for it to resurface afterwards.
Finally, continue to keep notes on Everything you tried out and discovered. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run issues and support Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, approach troubles methodically, and come to be simpler at uncovering concealed challenges in complicated techniques.
Produce Tests
Creating exams is one of the best tips on how to boost your debugging capabilities and In general development efficiency. Exams not merely enable capture bugs early but will also function a safety net that provides you self esteem when earning changes to your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically in which and when a dilemma takes place.
Get started with device assessments, which target particular person features or modules. These modest, isolated assessments can promptly expose no matter if a specific bit of logic is Performing as predicted. Every time a examination fails, you quickly know in which to search, substantially decreasing the time used debugging. Unit checks are In particular valuable for catching regression bugs—troubles that reappear right after previously remaining fastened.
Following, integrate integration checks and conclusion-to-conclude assessments into your workflow. These aid make sure that various aspects of your application function alongside one another efficiently. They’re specifically useful for catching bugs that come about in intricate systems with numerous factors or providers interacting. If something breaks, your assessments can let you know which Element of the pipeline failed and less than what problems.
Creating checks also forces you to Imagine critically regarding your code. To test a feature appropriately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of being familiar with In a natural way leads to higher code composition and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. After the take a look at fails consistently, it is possible to deal with fixing the bug and look at your check move when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from the disheartening guessing sport into a structured and predictable process—aiding you catch additional bugs, faster and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the challenge—observing your monitor for several hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new standpoint.
If you're much too near the code for much too long, cognitive exhaustion sets in. You might start overlooking obvious faults or misreading code that you wrote just several hours before. With this point out, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso split, and even switching to a special job for 10–quarter-hour can refresh your emphasis. Lots of builders report locating the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also enable avert burnout, Particularly during for a longer period debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping absent enables you to return with renewed energy and also a clearer attitude. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent rule of thumb is usually to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. click here Use that point to maneuver all around, stretch, or do a thing unrelated to code. It might experience counterintuitive, Specifically less than tight deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a sensible strategy. It offers your Mind space to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and rest is a component of resolving it.
Learn From Just about every Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you a thing important should you make the effort to replicate and review what went Incorrect.
Commence by asking by yourself some vital thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit screening, code opinions, or logging? The responses normally expose blind places as part of your workflow or knowledge and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be an outstanding routine. Hold a developer journal or keep a log where you Observe down bugs you’ve encountered, how you solved them, and Everything you realized. As time passes, you’ll start to see styles—recurring difficulties or widespread problems—which you can proactively stay away from.
In team environments, sharing what you've acquired from the bug using your peers can be Primarily strong. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. Rather than dreading bugs, you’ll get started appreciating them as vital parts of your progress journey. In any case, a lot of the ideal builders will not be those who compose fantastic code, but individuals who continuously understand from their mistakes.
In the long run, each bug you correct provides a brand new layer to your ability established. So next time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Conclusion
Bettering your debugging competencies requires time, exercise, and tolerance — however the payoff is big. It will make you a more effective, self-confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.